A state manager wrapper that allows redistribution of data across nodes. More...
#include <flat_state_mm_wrapper.h>
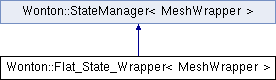
Public Types | |
using | pair_t = std::pair< std::string, Entity_kind > |
pair of name and entity to be used as data key More... | |
Public Member Functions | |
Flat_State_Wrapper (const MeshWrapper &mesh, std::unordered_map< std::string, int > const &names={}, std::unordered_map< int, std::vector< int >> const &material_cells={}) | |
Constructor for the state wrapper. More... | |
Flat_State_Wrapper & | operator= (Flat_State_Wrapper const &)=delete |
Assignment operator (disabled). More... | |
~Flat_State_Wrapper ()=default | |
Destructor. More... | |
template<class State_Wrapper > | |
void | initialize (State_Wrapper const &input, std::vector< std::string > const &var_names) |
Initialize the state wrapper with another state wrapper and a list of names. More... | |
size_t | get_num_vectors () |
Get the number of data vectors. More... | |
std::vector< double > | pack (std::string field_name) |
Turn the field into a vector of doubles. More... | |
void | unpack (std::string field_name, const std::vector< double > &flat_data, const std::vector< int > &all_material_ids={}, const std::vector< int > &all_material_shapes={}, const std::map< int, std::vector< int >> &distributedMaterialCellIds_={}) |
Turn a vector of doubles into the correct shape object. More... | |
size_t | get_field_stride (std::string field_name) |
Get field stride. More... | |
int | num_material_cells () const |
Return the number of material cells for this node. More... | |
std::vector< int > | get_material_ids () const |
Return the sorted vector of material ids actually used in the cell mat data. More... | |
std::vector< int > | get_material_shapes () const |
Return the sorted vector of material shapes actually used in the cell mat data. More... | |
std::vector< int > | get_material_cells () const |
Return the sorted vector of material shapes actually used in the cell mat data. More... | |
Flat_State_Wrapper ()=default | |
Constructor of Flat_State_Wrapper. More... | |
template<class State_Wrapper > | |
void | initialize (State_Wrapper const &input, std::vector< std::string > const &var_names) |
Initialize the state wrapper with another state wrapper and a list of names. More... | |
Flat_State_Wrapper & | operator= (Flat_State_Wrapper const &)=delete |
Assignment operator (disabled) - don't know how to implement (RVG) More... | |
~Flat_State_Wrapper ()=default | |
Empty destructor. More... | |
void | initialize (std::vector< std::string > const &names, std::vector< Entity_kind > const &entities, std::vector< std::shared_ptr< std::vector< T >>> const &data) |
Initialize the state wrapper with explicit lists of names, entities and data. More... | |
void | get_names (Entity_kind on_what, std::vector< std::string > &names) |
Get names of data fields associated with a given entity. More... | |
int | num_materials () const |
Number of materials in problem. More... | |
int | mat_get_num_cells (int matid) const |
Get number of cells containing a particular material. More... | |
void | mat_get_cells (int matid, std::vector< int > *matcells) const |
Get cell indices containing a particular material. More... | |
int | cell_get_num_mats (int cellid) const |
Get number of materials contained in a cell. More... | |
void | cell_get_mats (int cellid, std::vector< int > *cellmats) const |
Get the IDs of materials in a cell. More... | |
int | cell_index_in_material (int meshcell, int matid) const |
Get the local index of mesh cell in material cell list. More... | |
Field_type | field_type (Entity_kind on_what, std::string const &var_name) const |
Type of field (MESH_FIELD or MULTIMATERIAL_FIELD) More... | |
void | mesh_get_data (Entity_kind on_what, std::string const &var_name, T **data) |
Get pointer to scalar data. More... | |
void | mesh_get_data (Entity_kind on_what, std::string const &var_name, T const **data) const |
Get pointer to scalar data. More... | |
void | mat_get_celldata (std::string const &var_name, int matid, T const **data) const |
Get pointer to read-only scalar cell data for a particular material. More... | |
void | mat_get_celldata (std::string const &var_name, int matid, T **data) |
Get pointer to read-write scalar data for a particular material. More... | |
Entity_kind | get_entity (std::string const &var_name) const |
Get the entity type on which the given field is defined. More... | |
Entity_kind | get_entity (int index) const |
Get the entity type on which the given field is defined. More... | |
size_t | get_entity_size (Entity_kind ent) |
Get size for entity. More... | |
const std::type_info & | get_data_type (std::string const &name) const |
Get the data type of the given field. More... | |
size_t | get_vector_index (Entity_kind ent, std::string const &name) |
Get index for entity and name. More... | |
std::shared_ptr< std::vector< T > > | get_vector (size_t index) |
Get the data vector. More... | |
std::shared_ptr< std::vector< Wonton::Point3 > > | get_gradients (size_t index) |
Get gradients. More... | |
size_t | get_num_vectors () |
Get the number of data vectors. More... | |
void | add_gradients (std::shared_ptr< std::vector< Wonton::Point3 >> new_grad) |
Add a gradient field. More... | |
size_t | get_field_stride (size_t index) |
Get field stride. More... | |
size_t | get_num_gradients () |
Get the number of gradient vectors. More... | |
![]() | |
StateManager (const MeshWrapper &mesh, std::unordered_map< std::string, int > names={}, std::unordered_map< int, std::vector< int >> material_cells={}) | |
Constructor. More... | |
void | add_material_names (const std::unordered_map< std::string, int > &names) |
Add the names of the materials. More... | |
std::vector< std::string > const | names () const |
Return the names registered by the state manager. More... | |
std::string | material_name (int m) const |
Return the name of a material from its material id. More... | |
int | get_material_id (std::string const &name) const |
Return the material id from its name. More... | |
void | add_material_cells (std::unordered_map< int, std::vector< int >> cells) |
Add the material cells. More... | |
std::unordered_map< int, std::vector< int > > const & | get_material_cells () const |
Return the material cells. More... | |
std::unordered_map< int, std::unordered_set< int > > const & | get_cell_materials () const |
Return the cell materials. More... | |
std::unordered_map< int, int > const | get_material_shape () const |
Return the number of cells for each material. More... | |
int | num_materials () const |
Return the number of materials in the problem. More... | |
Entity_kind | get_entity (std::string const &name) const |
Return the entity kind for a state vector. More... | |
Field_type | field_type (Entity_kind kind, std::string const &name) const |
Return the field type of a state vector. More... | |
int | get_data_size (Entity_kind on_what, std::string const &name) const |
Return the data size . More... | |
const std::type_info & | get_data_type (std::string var_name) |
Get the data type of the given field. More... | |
void | add (std::shared_ptr< StateVectorBase > sv) |
Add a StateVectorBase to the state manager. More... | |
template<class T > | |
void | add (std::shared_ptr< StateVectorUni< T >> sv) |
Add a StateVectorUni<T> to the state manager. More... | |
template<class T > | |
void | add (std::shared_ptr< StateVectorMulti< T >> sv) |
Add a StateVectorMulti<T> to the state manager. More... | |
std::shared_ptr< StateVectorBase > | get (std::string name) |
Get a shared pointer to a StateVectorBase from the state manager. More... | |
std::shared_ptr< StateVectorBase const > | get (std::string name) const |
Get a shared pointer to a const StateVectorBase from the state manager. More... | |
template<typename T > | |
std::shared_ptr< T > | get (std::string name) |
Get a shared pointer to a templated type from the state manager. More... | |
template<typename T > | |
std::shared_ptr< T > | get (std::string name) const |
Get a shared pointer to a templated type from the state manager. More... | |
int | num_material_cells (int m) const |
Return the number of cells for this material id. More... | |
std::vector< int > const & | get_material_cells (int m) const |
Return the cell ids for this material id. More... | |
void | mat_get_cells (int m, std::vector< int > *matcells) const |
Return the cell ids for this material id. More... | |
template<class T > | |
void | mat_get_celldata (std::string const &name, int m, T const **data) const |
Return the cell data for this material id. More... | |
int | cell_index_in_material (int c, int m) const |
Return the cell index in this material id. More... | |
template<class T > | |
void | mat_get_celldata (std::string const &name, int m, T **data) |
Return the cell data for this material id. More... | |
int | cell_get_num_mats (int c) const |
Return the number of materials in this cell. More... | |
std::unordered_set< int > | get_cell_materials (int c) const |
Return the unordered set of materials in this cell. More... | |
void | cell_get_mats (int c, std::vector< int > *cellmats) const |
Return the materials in this cell. More... | |
template<class T > | |
void | mesh_get_data (Entity_kind on_what, std::string const &name, T const **data) const |
Return the const cell data for this material id. More... | |
template<class T > | |
void | mesh_get_data (Entity_kind on_what, std::string const &name, T **data) |
Return the cell data for this material id. More... | |
void | mat_add_cells (int m, std::vector< int > const &newcells) |
Add cells for a new material to the material cells. More... | |
template<class T > | |
void | mat_add_celldata (std::string const &name, int m, T const *values) |
Add cell data for a new material in a state vector to the state manager. More... | |
void | add_material (std::string const &matname, std::vector< int > const &matcells) |
Add cell data for a new material in a state vector to the state manager. More... | |
template<class T = double> | |
bool | shape_is_good (const std::shared_ptr< StateVectorMulti< T >> sv) |
Check that a StateVectorMulti<T> has a shape consistent with the state manager. More... | |
void | clear_material_cells () |
Clear material cells and the inverse map of cell materials. More... | |
Additional Inherited Members | |
![]() | |
void | clear () |
![]() | |
const MeshWrapper & | mesh_ |
std::unordered_map< std::string, std::shared_ptr< StateVectorBase > > | state_vectors_ |
std::unordered_map< std::string, int > | material_ids_ |
std::unordered_map< int, std::string > | material_names_ |
std::unordered_map< int, std::vector< int > > | material_cells_ |
std::unordered_map< int, std::unordered_map< int, int > > | cell_index_in_mat_ |
std::unordered_map< int, std::unordered_set< int > > | cell_materials_ |
Detailed Description
template<class MeshWrapper>
class Wonton::Flat_State_Wrapper< MeshWrapper >
A state manager wrapper that allows redistribution of data across nodes.
Stores state data in a flat representation.
Currently all fields must be of the same type
Member Typedef Documentation
◆ pair_t
using Wonton::Flat_State_Wrapper< MeshWrapper >::pair_t = std::pair<std::string, Entity_kind> |
pair of name and entity to be used as data key
Constructor & Destructor Documentation
◆ Flat_State_Wrapper() [1/2]
|
inlineexplicit |
Constructor for the state wrapper.
- Parameters
-
[in] mesh mesh wrapper [in] names map from material names to material id [in] material_cells map from material id to vector of cells
Constructor that takes the meshwrapper for the underlying mesh and two optional map arguments: the map from material name to id, and the map from material id to the cells containing that material
◆ ~Flat_State_Wrapper() [1/2]
|
default |
Destructor.
◆ Flat_State_Wrapper() [2/2]
|
default |
Constructor of Flat_State_Wrapper.
◆ ~Flat_State_Wrapper() [2/2]
|
default |
Empty destructor.
Member Function Documentation
◆ add_gradients()
|
inline |
Add a gradient field.
◆ cell_get_mats()
|
inline |
Get the IDs of materials in a cell.
- Parameters
-
cellid Index of cell in mesh cellmats Indices of materials in cell
◆ cell_get_num_mats()
|
inline |
Get number of materials contained in a cell.
- Parameters
-
cellid Index of cell in mesh
- Returns
- Number of materials in cell
◆ cell_index_in_material()
|
inline |
Get the local index of mesh cell in material cell list.
- Parameters
-
meshcell Mesh cell ID matid Material ID
- Returns
- Local cell index in material cell list
◆ field_type()
|
inline |
Type of field (MESH_FIELD or MULTIMATERIAL_FIELD)
- Parameters
-
onwhat Entity_kind that field is defined on varname Name of field
- Returns
- Field type
◆ get_data_type()
|
inline |
Get the data type of the given field.
- Parameters
-
[in] name The string name of the data field
- Returns
- A reference to the type_info struct for the field's data type
◆ get_entity() [1/2]
|
inline |
Get the entity type on which the given field is defined.
- Parameters
-
[in] var_name The name of the data field
- Returns
- The Entity_kind enum for the entity type on which the field is defined
If the name has previously been associated with more than one entity, then the entity that was most recently associated will be returned. To avoid this ambiguity, please provide entity hints in the field name for yourself.
This function is provided to make the class compatible with other state wrappers.
◆ get_entity() [2/2]
|
inline |
Get the entity type on which the given field is defined.
- Parameters
-
[in] index The index of the data field
- Returns
- The Entity_kind enum for the entity type on which the field is defined
◆ get_entity_size()
|
inline |
Get size for entity.
◆ get_field_stride() [1/2]
|
inline |
Get field stride.
◆ get_field_stride() [2/2]
|
inline |
Get field stride.
◆ get_gradients()
|
inline |
Get gradients.
◆ get_material_cells()
|
inline |
Return the sorted vector of material shapes actually used in the cell mat data.
- Returns
- vector<int> of material shapes (ordered)
Return the sorted vector of material shapes actually used in the cell mat data.
◆ get_material_ids()
|
inline |
Return the sorted vector of material ids actually used in the cell mat data.
- Returns
- vector<int> of material id's (ordered)
Return the sorted vector of material ids actually used in the cell mat data.
◆ get_material_shapes()
|
inline |
Return the sorted vector of material shapes actually used in the cell mat data.
- Returns
- vector<int> of material shapes (ordered)
Return the sorted vector of material shapes actually used in the cell mat data.
◆ get_names()
|
inline |
Get names of data fields associated with a given entity.
- Parameters
-
[in] entity The entity type [out] names The names associated with the entity. Cleared on entry.
◆ get_num_gradients()
|
inline |
Get the number of gradient vectors.
◆ get_num_vectors() [1/2]
|
inline |
Get the number of data vectors.
- Returns
- The number of state vectors
◆ get_num_vectors() [2/2]
|
inline |
Get the number of data vectors.
◆ get_vector()
|
inline |
Get the data vector.
◆ get_vector_index()
|
inline |
Get index for entity and name.
◆ initialize() [1/3]
|
inline |
Initialize the state wrapper with another state wrapper and a list of names.
- Parameters
-
[in] input another state wrapper, which need not be for Flat_State. [in] var_names a list of state names to initialize
Entities and sizes associated with the given name will be obtained from the input state wrapper.
A name can be re-used with a different entity, but a name-entity combination must be unique.
A name-entity combination must not introduce a new size for that entity if it has previously been encountered.
All existing internal data is forgotten.
◆ initialize() [2/3]
|
inline |
Initialize the state wrapper with another state wrapper and a list of names.
- Parameters
-
[in] input another state wrapper, which need not be for Flat_State. [in] var_names a list of state names to initialize
Entities and sizes associated with the given name will be obtained from the input state wrapper.
A name can be re-used with a different entity, but a name-entity combination must be unique.
A name-entity combination must not introduce a new size for that entity if it has previously been encountered.
All existing internal data is forgotten.
◆ initialize() [3/3]
|
inline |
Initialize the state wrapper with explicit lists of names, entities and data.
- Parameters
-
[in] names a list of state names to initialize [in] entities entities corresponding to the names [in] data the state vectors to be stored
A name can be re-used with a different entity, but a name-entity combination must be unique.
A name-entity combination must not introduce a new size for that entity if the entity has previously been encountered.
All existing internal data is forgotten.
◆ mat_get_celldata() [1/2]
|
inline |
Get pointer to read-only scalar cell data for a particular material.
- Parameters
-
[in] var_name The string name of the data field [in] matid Index (not unique identifier) of the material [out] data vector containing the values corresponding to cells in the material
◆ mat_get_celldata() [2/2]
|
inline |
Get pointer to read-write scalar data for a particular material.
- Parameters
-
[in] on_what The entity type on which to get the data [in] var_name The string name of the data field [in] matid Index (not unique identifier) of the material [out] data vector containing the values corresponding to cells in the material
Removing the constness of the template parameter allows us to call this function and get const data back (e.g. pointer to double const) even if the wrapper object is not const. The alternative is to make another overloaded operator that is non-const but returns a pointer to const data. Thanks StackOverflow!
◆ mat_get_cells()
|
inline |
Get cell indices containing a particular material.
- Parameters
-
matid Index of material (0, num_materials()-1) matcells Cells containing material 'matid'
◆ mat_get_num_cells()
|
inline |
Get number of cells containing a particular material.
- Parameters
-
matid Index of material (0, num_materials()-1)
- Returns
- Number of cells containing material 'matid'
◆ mesh_get_data() [1/2]
|
inline |
Get pointer to scalar data.
- Parameters
-
[in] on_what The entity type on which the data is defined [in] var_name The string name of the data field [in,out] data A pointer to an array of data. Null on output if data does not exist.
Data is associated with the name-entity combination. Both values must be valid.
◆ mesh_get_data() [2/2]
|
inline |
Get pointer to scalar data.
- Parameters
-
[in] on_what The entity type on which the data is defined [in] var_name The string name of the data field [in,out] data A pointer to an array of data. Null on output if data does not exist.
Data is associated with the name-entity combination. Both values must be valid.
◆ num_material_cells()
|
inline |
Return the number of material cells for this node.
- Returns
- number of material cells for this node
Return the number of material cells for this node. This number is the sum of the lengths of the material cell indices vectors. It is the number need to pass a flattened state vector.
◆ num_materials()
|
inline |
Number of materials in problem.
◆ operator=() [1/2]
|
delete |
Assignment operator (disabled).
◆ operator=() [2/2]
|
delete |
Assignment operator (disabled) - don't know how to implement (RVG)
◆ pack()
|
inline |
Turn the field into a vector of doubles.
- Parameters
-
[in] field_name The field name
- Returns
- The serialized vector of doubles
◆ unpack()
|
inline |
Turn a vector of doubles into the correct shape object.
- Parameters
-
[in] field_name The field name [in] flat_data The serialized striped vector of doubles to be unpacked [in] all_material_ids The striped material ids from all nodes [in] all_material_shapes The striped material shapes from all nodes
The documentation for this class was generated from the following files:
- /home/wonton/wonton/wonton/state/flat/flat_state_mm_wrapper.h
- /home/wonton/wonton/wonton/state/flat/flat_state_wrapper.h