Parts mismatch fixup tests for part-by-part interpolation. More...
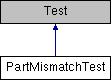
Public Member Functions | |
PartMismatchTest () | |
Setup each test-case. More... | |
void | unitTest (Partial partial_fixup, Empty empty_fixup) |
Do the test. More... | |
Protected Types | |
using | Remapper = Portage::CoreDriver< 2, Wonton::Entity_kind::CELL, Wonton::Jali_Mesh_Wrapper, Wonton::Jali_State_Wrapper > |
using | PartPair = Portage::PartPair< 2, Wonton::Jali_Mesh_Wrapper, Wonton::Jali_State_Wrapper > |
using | Partial = Portage::Partial_fixup_type |
using | Empty = Portage::Empty_fixup_type |
Protected Member Functions | |
double | compute_source_density (int c) |
Compute source cell density. More... | |
template<bool is_source> | |
void | create_partition (double thresh) |
Create a partition based on a threshold value. More... | |
double | get_expected_remapped_density (int const cell, Partial partial_fixup, Empty empty_fixup) |
Compute the expected remapped density on target mesh. More... | |
Protected Attributes | |
std::shared_ptr< Jali::Mesh > | source_mesh |
std::shared_ptr< Jali::Mesh > | target_mesh |
std::shared_ptr< Jali::State > | source_state |
std::shared_ptr< Jali::State > | target_state |
Wonton::Jali_Mesh_Wrapper | source_mesh_wrapper |
Wonton::Jali_Mesh_Wrapper | target_mesh_wrapper |
Wonton::Jali_State_Wrapper | source_state_wrapper |
Wonton::Jali_State_Wrapper | target_state_wrapper |
std::vector< PartPair > | parts |
std::vector< int > | source_cells [nb_parts] |
std::vector< int > | target_cells [nb_parts] |
Static Protected Attributes | |
static constexpr int const | nb_parts = 2 |
static constexpr double | upper_bound = std::numeric_limits<double>::max() |
static constexpr double | lower_bound = std::numeric_limits<double>::min() |
static constexpr double | epsilon = 1.E-10 |
Detailed Description
Parts mismatch fixup tests for part-by-part interpolation.
Here, the purpose is to check that parts mismatch are correctly handled when interpolating each pair of source-target part independently. More precisely, we aim to ensure that values within partially filled and empty cells are correctly fixed according to the user-specified fixup scheme which may be:
- constant and leave empty,
- constant and extrapolate,
- locally conservative and leave empty,
- locally conservative and extrapolate,
- shifted conservative and leave empty,
- shifted conservative and extrapolate.
Again, source and target meshes are respectively split into two parts, and each source-target part pair is remapped independently. Remapped values are then compared to the exact expected values.
0,1 source 1,1 ___________________ source mesh: 4x4 cartesian grid. | | : | | Here, the domain is halved into two equal parts. |____|____:____|____| The prescribed density is 100 for the first part | | : | | and 1 for the second part. |____0____:____1____| | | : | |
____ | ____:____ | ____ |
---|---|---|
____ | ____:____ | ____ |
___________________ target mesh: 5x5 cartesian grid. | | | . | : | Here parts interface is shifted such that the |___|___|_._|___:___| first part is 4/5 of the domain and the last | | | . | : | part is only 1/5 of it. |___|___0_._|___:1| Hence, we have a: | | | . | : | - partially filled cells layer in [0.5-0.6] |___|___|_._|___:___| - empty cells layer in [0.6-0.8] | | | . | : | |___|___|_._|___:___|
0,0 target 1,0
Member Typedef Documentation
◆ Empty
|
protected |
◆ Partial
|
protected |
◆ PartPair
|
protected |
◆ Remapper
|
protected |
Constructor & Destructor Documentation
◆ PartMismatchTest()
|
inline |
Setup each test-case.
It creates both source and target meshes, then computes and assigns a density field on source mesh, then creates parts couples for both source and target meshes.
Member Function Documentation
◆ compute_source_density()
|
inlineprotected |
Compute source cell density.
- Parameters
-
c the source cell local index
- Returns
- the exact density of the given cell.
◆ create_partition()
|
inlineprotected |
Create a partition based on a threshold value.
- Template Parameters
-
is_source source or target mesh?
- Parameters
-
thresh x-axis threshold value
◆ get_expected_remapped_density()
|
inlineprotected |
Compute the expected remapped density on target mesh.
It depends on Partial/empty mismatch fixup schemes being used:
- locally-conservative/leave-empty: [100.0|100.0| 50.0| 0.0|1.0]
- locally-conservative/extrapolate: [100.0|100.0| 50.0| 50.0|1.0]
- constant/leave-empty: [100.0|100.0|100.0| 0.0|1.0]
- constant/extrapolate: [100.0|100.0|100.0|100.0|1.0]
- shifted-conservative/leave-empty: [ 83.3| 83.3| 83.3| 0.0|2.5]
- shifted-conservative/extrapolate: [ 62.5| 62.5| 62.5| 62.5|2.5]
- Parameters
-
cell the target cell local index partial_fixup the Partial mismatch fixup scheme to use empty_fixup the empty cell fixup scheme to use
- Returns
- the expected remapped density value
◆ unitTest()
Do the test.
- Parameters
-
partial_fixup the partially filled cell fixup scheme to use. empty_fixup the empty cells fixup scheme to use.
Member Data Documentation
◆ epsilon
|
staticprotected |
◆ lower_bound
|
staticprotected |
◆ nb_parts
|
staticprotected |
◆ parts
|
protected |
◆ source_cells
|
protected |
◆ source_mesh
|
protected |
◆ source_mesh_wrapper
|
protected |
◆ source_state
|
protected |
◆ source_state_wrapper
|
protected |
◆ target_cells
|
protected |
◆ target_mesh
|
protected |
◆ target_mesh_wrapper
|
protected |
◆ target_state
|
protected |
◆ target_state_wrapper
|
protected |
◆ upper_bound
|
staticprotected |
The documentation for this class was generated from the following file:
- /home/portage/portage/portage/driver/test/test_driver_part_mismatch.cc